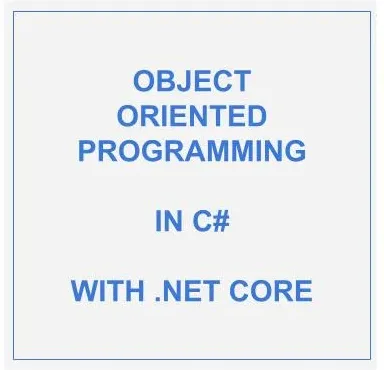
This is a complete tutorial for Object Oriented Programming in C# with .NET CORE. In this tutorial, you will learn about OOP concepts, different pillars of the language, building blocks (Classes and Objects), and how we can design a program that is primarily oriented toward Objects.
PILLARS OF OBJECT ORIENTED PROGRAMMING
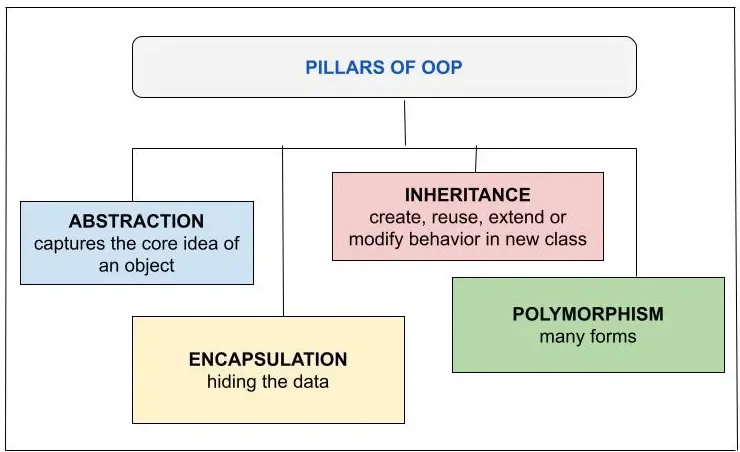
C# Classes
- A class defines a type of object
Ex: Student, Staff, Course - It is used to store data & take actions on them
- Classes are declared by using the “class” keyword
Eg:class Student { }
- A class should have one responsibility.
- Extending a type is called Classes.
C# Objects
- An Object is a concrete entity based on a class.
- An Object refers to an instance of a class.
- Objects are created using “new” keyword
Eg:Student obj = new Student();
Constructors
They are methods that are called when object is created or instantiated.
They are defined within the class & has the class name in their declaration.
Eg:
public class Student { //constructor public Student() { //All initialization goes here } }
Abstraction
- Abstraction is generally a concept.
- Understand the underlying concept rather than the definition.
- It is about capturing the core idea of an object & ignoring the details.
- It is achieved by exposing the essential features of the entity by hiding the other irrelevant details.
Why do we need Abstraction ?
- It reduces complexity
- increases efficiency
C# Class Fields
C# Fields
- Fields are used to store data in a type.
Eg:
Student Class
-FirstName : string
-LastName : string
-ID : int
-Age : int
- Defined by : Access Level + Type + Name
Eg:
public class Student { public string FirstName; public string LastName; public int ID; public int Age; }
- Fields are accessed using . (dot) symbol
Eg:Student.FirstName
- Fields are initialized immediately before the constructor for the object instance is called.
- If constructor assigns value of a field, it will override any value given during field declaration..
Types of C# Fields
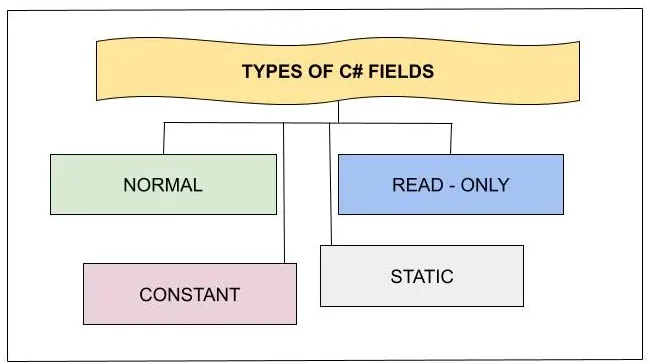
Read-Only
- Except in Constructor, their value can’t be changed.Eg:
int readonly maxStudents = 120;
Constant
- Their value can’t be changed
Eg:int const maxStudents = 120;
COMPARE READ-ONLY & CONSTANT FIELDS IN C#
READ – ONLY | CONSTANT | |
Assign value during compile time | ✅ | ✅ |
Assign value during runtime | ✅ | ❎ |
Dependent assemblies don’t need to re-build | ✅ | ❎ |
More recommended | ✅ | ❎ |
Static Fields
- Static fields are shared across all instances of a class.
Eg:public static int MaxAllowedStudents;
- Field name is prefixed with the keyword “static” to mark it as a static field.
Eg:public static int MaxEnrolledStudents;
- Static member is always accessed by the class name, not the instance name.
Eg:Student.MaxEnrolledStudent;
- Only one copy of a static member exists; regardless of how many instances of classes has been created.
Value Types versus Reference Types
VALUE TYPES | REFERENCE TYPES |
Variable Contains | |
Instance of the Type | Reference to the instance of the Type |
When you make changes | |
Changes are applied only for this instance | Changes are applied to both the instances |
Eg: CHAR, INT, FLOAT, BOOL | Eg: STRING, OBJECT, CLASS |
The Reference Type holds the address to the instance | |
|
|
Access Modifiers In C#
C# Supports 6 Access Modifiers
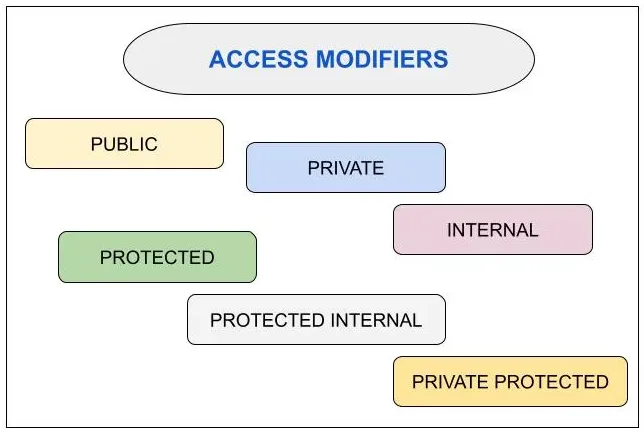
PUBLIC
- Accessible by any type.
- There are no restrictions on their usage.
- Typically, class types are marked as public.
PRIVATE
- Accessible only within the type.
- If we define FirstName as private field to the Student Class, then that field can be used only within that class, no other type can access it.
- Typically, the class fields are marked as private.
INTERNALS
- Accessible by any type within the current assembly
- i.e. any type within the assembly can assess it.
- It is typically used to define dependent types that are not useful for other assemblies.
NOTE:
BY DEFAULT, CLASS IS CONSIDERED INTERNAL AND CLASS MEMBERS ARE PRIVATE.
C# Class Methods
In C#, we need methods to take actions over fields.
C# Methods
- A Method is a code block that contains a series of statements.
- Methods are used to take actions on a type’s fields
- They are also referred to as functions.
Eg:
A student can enroll in a course is stored in a field within Student Class.
In order to assign a course to this field, user can add a method “Enroll” in a Student Class.
public void Enroll (Course newCourse)
{
course = newCourse
}
- A Class Method has a
– a name
– return type &
– a list of parameters
– followed by the method body
– Access Modifier is optional; default it is considered “PRIVATE” - Each Method can return only one type.
- To return more than one type, we can use “Tuples” which is combination of more than one type.
Method Signature
Method Name + Parameter Types + Return Type + Access Modifier
Type Of Methods A Class Can Have
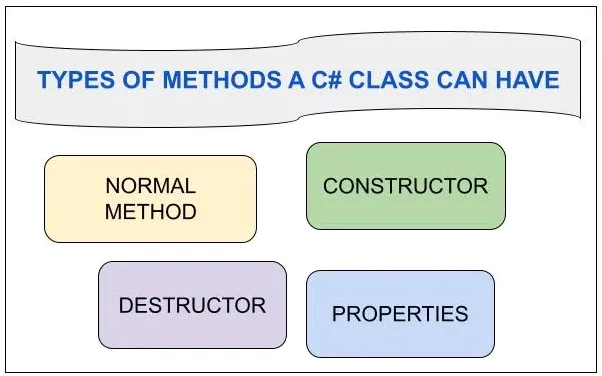
NORMAL METHODS
Example:
public class Student
{
public int Id;
public string firstName;
public string lastName;
public int GetID()
{
return Id;
}
public string GetFullName()
{
return $ "{firstName} {lastName}";
}
}
public class Program
{
Student student1 = new Student()
{
firstName = "Hira",
lastName = "Lal",
Id = 101
};
Console.WriteLine(student1.GetId());
Console.WriteLine(student1.GetFullName());
}
Output:
101
Hira Lal
CONSTRUCTORS
- Constructors are methods that get called when an object is instantiated using “new” keyword.
- They are used to initialize class members along with any other initialization.
Eg: Student class constructor
public Student()
{
Console.WriteLine("In Constructor");
}
- Constructors does not have return type.
- If fields in constructor are defied with default, they can be initialized before the call for constructor.
- We can have multiple constructors for a class, based on type. Depending on way the object is instantiated, specific/appropriate constructor will get called.
DESTRUCTORS
- Destructors are methods that get called when a class instance is being collected by the garbage collector.
- They are used to perform final clean-up operations (if required).
- It is optional to define a destructor for a type
Eg:
~Student()
{
// Handle cleanup operations here.
}
NOTE:
Destructors does not have access modifiers, return types as well as parameters.
Destructors are also called FINALIZERS.
COMPARISON BETWEEN CONSTRUCTORS V/S DESTRUCTORS
CONSTRUCTORS | DESTRUCTORS | |
Gets called during initialization | ✅ | ❎ |
Gets called during Garbage Collection | ❎ | ✅ |
Can be invoked manually | ✅ | ❎ |
Can take access modifiers & params | ✅ | ❎ |
Multiple Methods Possible | ✅ | ❎ |
PROPERTIES
- Property is a method that looks like a field to get & set a value.
- It’s a kind of hybrid of fields & methods. It looks like a field during usage, but its a method.
- C# Property has 3 Parts
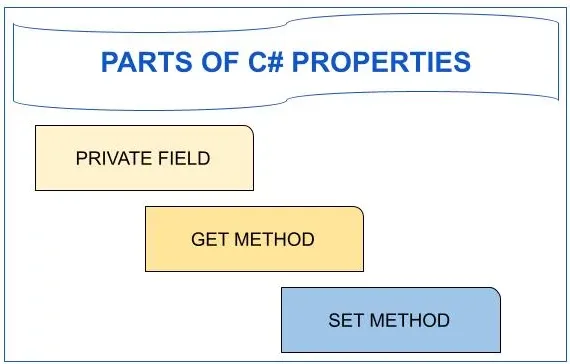
private string firstName;
public string FirstName
{
get
{
return firstName;
}
set
{
firstName = value;
}
}
- C# supports auto-property for all the 3 parts & they are implicitly defined.
- An auto-property is a method with get & set implicitly defined.
Eg:
public string firstName
{
get;
set;
}
- Example for Auto-property with default value
public string firstName {get; set;} = string.Empty;
- A property with only get; is called Read-Only property.
ENCAPSULATION
- It refers to hiding all private data & exposing them only through properties or methods.
- This avoids exposing the inner details of the class/type to the outer world.
- The TYPE encapsulate all details and outside world only needs to refer to a type in broader sense.
WHY IS ENCAPSULATION REQUIRED ?
Protects data.
Easy to manage.
Control who can access actions & data.
HOW TO ACHIEVE IT ?
Marking all fields as PRIVATE.
Allowing access only through properties & methods.
Access modifiers.
- Example:
public class Course
{
public int CourseId;
public string CourseName;
public static int MaxSubjects = 8;
private List<CourseSubject> Subjects;
public List<CourseSubject> Subjects
{
get
{
return subjects;
}
private set;
}
}
Types Of Passing Parameters
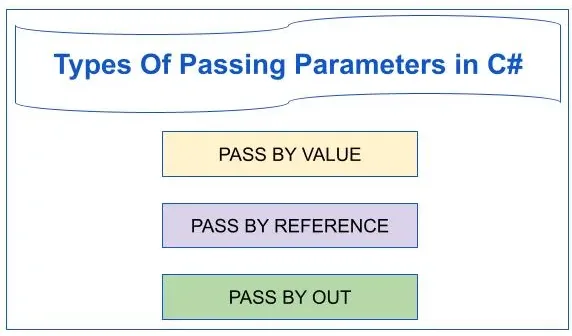
PASS BY VALUE
- Value of the variable is passed. (ONLY IN).
PASS BY REFERENCE
- A reference to the variable is passed.
i.e. the address of the variable (IN & OUT)
PASS AS OUT PARAMETER
- Any incoming value is ignored. (ONLY OUT)
- It is mandatory for its parameter to be assigned a value inside the method before he control is returned.
- Eg:
public void Calculator (decimal var1, ref decimal var2, out decimal var3)
{
var1 = 20.5;
var2 = 29.5;
var3 = var1 + var2;
}
Method Overloading
- Method Overloading refers to having more than one method definition for a method name, but with a different signature.
- Eg:
public void UpdateInfo (string firstName)
{
FirstName = firstName;
}
public void UpdateInfo (string firstName, string lastName)
{
FirstName = FirstName;
LastName = lastName;
}
//Method Overloading
obj.UpdateInfo ("Raj");
obj.UpdateInfo ("Raj", "Kumar");
Optional Parameters
- Instead of overloading, we can make the method parameters as Optional.
- Eg:
public void UpdateInfo (string firstName, string lastName = "")
{
//body goes here...
}
- Assign the parameters with default values to make them Optional.
- Default value assigned must be a literal value.
Eg:
(a)Empty string literal in our previous example.
(b) Or a numeric value for an int parameter
& so on…. - They can only (always) appear as last in the list of parameters.
- Also can refer to the parameters by their name.
Static Classes
- Static class is a class that cannot be instantiated.
- We can still refer to class members without its instance creation.
- They are mostly used for utility classes which don’t require instance for each user.
Eg: Display, logger, etc… - Keyword “static” must be used in the class definition
- All members of static class must be declared “static” as well.
Reason is, since the static class cannot have instance, there is no way of having no static defining members
Advantages of Static Classes
- Easy to maintain – As no separate instance of this class is possible.
- Saves development and testing time.
- Reduces bugs.
C# INTERFACES
- An interface contains definition for a group of related functionalities that a non-abstract class or a struct must implement.
- If a type implements an interface then it promises it supports certain features.
- An interface is a way to define a contract.
i.e. if a class implements an interface that means it has all methods & properties defined in a contract. - We can define an interface by using the “Interface” keyword.
- Eg:
public interface IStudent
{
//interface members goes here...
}
Interface Members
- It can be
(a) Methods,
(b) Properties,
(c) Events,
(d) Indexers,
OR any combinations of all. - An interface cannot declare instance data members such as fields.
- An interface can’t be instantiated directly.
- It must be implemented by a class/struct that implements all the interface members.
- A class/struct must provide an implementation for all of the members that the interface declares.
- To implement an interface member, the corresponding member of the implementing class must be public, non-static and have the same name & signature of the base member.
Implementing an Interface
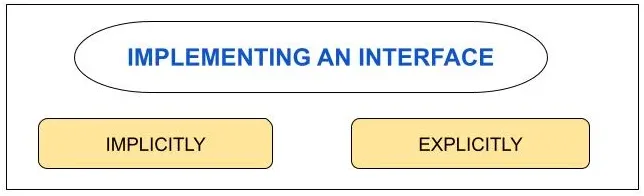
Implicit Interface
- The interface members are defined just like a normal method without any reference to an interface name.
- This is a default behavior where an interface is implemented using a class.
- The method must be public in such a case.
Explicit Interface
- The name of the interface is prefixed in each of the interface member implementations.
- Explicitly implemented interfaces don’t have public access modifiers.
Default Interface Implementation
- Starting C# 8.0, we can have default implementation of methods in an interface.
- The default implementation is very useful for interface that add a new method in the future.
- It is supported for members & properties as well.
- The only condition is, properties with default implementation can acess only the fileds that are within the interface definition.
- We cannot assign this to an auto-property within an interface.
Eg:
public interface ICourse
{
int TotalSubjects
{
get
{
return Subjects.Count();
}
}
List <CourseSubject> Subjects { get; }
}
Properties in Interface
- Properties are methods
- Example for properties with an implicit backing field
public interface ICourse
{
int TotalDays { get; set; }
}
- Just like any other method this property needs to be implemented in class.
- You can implement either with the auto property OR full property with get & set;
- Eg:
public int TotalDays
{
get
{
//
}
set
{
//
}
}
Static Members In Interface
- An interface may contain static fields and methods.
- In such a case, they belong to the interface & not to the class.
- Eg:
public interface ICourse
{
static int DefaultCout = 8;
}
- To refer in class…
public class Student : ICourse
{
ICourse.DefaultCount = 10;
}
- We can also define static member similar way…
pubic interface ICourse
{
static void ShowCount()
{
//Define implementations
}
}
- Static Members in an interface must be implemented within the interface.
Why are Interfaces required ? (its uses)
- Establish a contract that needs to be honored by all types implementing it.
This provides blue print of method that guarantees to be present & available for use by any type that implements that interface. - We can swap the type assigned to an interface object without breaking existing code.
As long as the type honors the interface contract by implementing the mentioned members, then it can be passed through an interface object. - By using interfaces, we can include behavior form multiple sources in a class.
- Since, C# does not support multiple inheritance of classes, we can achieve this functionality through the use of interface.
- Interfaces helps to reduce coupling.
Therefore allows you to easily inter-change the underlying concept without the code be affected. - We can use interface to simulate inheritance for structs.
Because they cannot actually inherit from another struct or class.
C# INHERITANCE
- Inheritance enables you to create new classes that reuse, extend & modify the behavior defined in other classes.
- The class from where we inherit the members are called Base Class.
- And the class that inherits from those base classes are called Derived Class.
Eg:
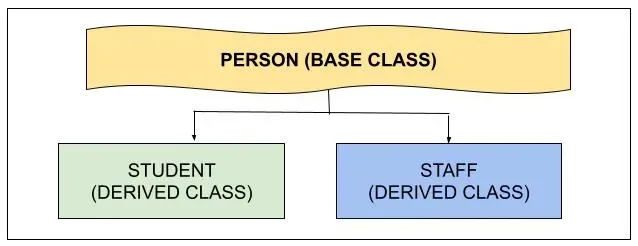
- We can add more members in the derived class to extend the functionality of the base class.
- Derived class constructor can internally call the base class constructor using base();
- The base class constructor gets called first followed by the derived class.
- When we don’t call the base class constructor, the system will internally call the base class’s default constructor.
- Default Constructor is created implicitly only when no other constructors are defined within the type.
Access Modifier: PROTECTED
Accessible with-in the type & any type derived from it.
Access Modifier: PRIVATE PROTECTED
Accessible within the type OR any type derived from it with-in the current assembly.
Access Modifier: PROTECTED INTERNAL
Accessible by any type with-i the current assembly OR any type derived from it.
Access Levels
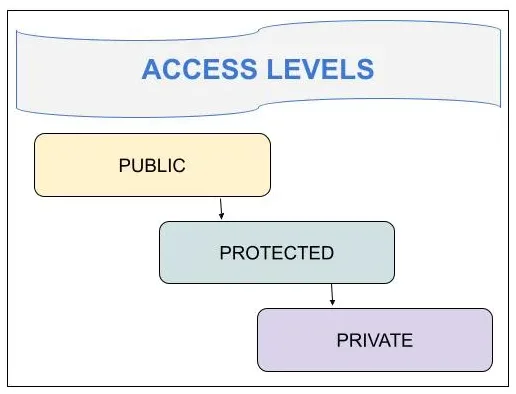
Casting in Inheritance
If the objects assigned are of different types, then casting is required. There are 2 types.
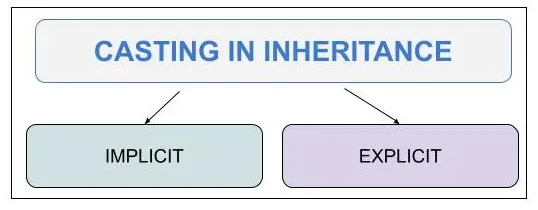
Implicit Casting
- If an instance of a derived type is assigned to that of a base type then Implicit Casting is done.
- No manual casting is required.
- Eg:
Person person = new Student (1, "Raj", "Kumar");
Console.WriteLine(Person.GetFullName());
Explicit Casting
- An instance of base type is assigned to that of a derived type instance.
- Manual casting must be done in this case.
- Eg:
List<String> FullName = ((Student)Person).Hobbies;
- To use Explicit casting, use the “as” operator to try converting to derived type.
- Eg:
List<String> FullName = (person as Student).Hobbies;
//will return null if could not cast...
- For better Explicit casting, you can also use “is” operator to first check whether the instance is of derived type and then case it.
- Eg: (1)
Student stud1 = person as Student;
if(stud1 !=null)
{
// implementation here...
}
- Eg: (2)
if (person is Student)
{
//Refer Student members here...
Student stud1 = person as Student;
List<string>Hobbies = stud1.Hobbies;
}
Why Multiple Inheritance is not allowed in C# ?
- ln C#, only single inheritance is allowed.
- Adds more complexity for the overall design & implementation.
- Adds only little benefit to the language.
- Need to support in other .NET Languages such as VB.NET.
POLYMORPHISM
- “Poly” means many & “morph” means forms. Hence, it means many forms.
- Polymorphism refers to the ability to take multiple forms hat allows us to invoke derived class methods through a base class reference during run time.
- It allows a derived class to override an inherited action to provide custom behavior.
- It is achieved by marking the methods with the “virtual” keyword.
- Eg:
public virtual void AddSubject (Course course)
{
//implementation goes here...
}
NOTE: The class fields cannot be virtual.
- Expose class fields through properties to make them virtual.
Method Hiding
- It allows a derived type to define the same method present in the base class with a different implementation & hide it from the Base Class.
- Add “new” Keyword to the derived class’s method implementation to hide it from the base class.
- The method defined in the derived class using the “new” keyword doesn’t participate in polymorphism.
Method Overriding
- Override the method in the derived type using the “override” keyword.
- The Base Class method must be defined using the “virtual” keyword.
- Any class inheriting a virtual method can implement it’s own version.
- The Base class method can be called from with-in the derived class using the “base” keyword.
- Eg:
base MethodName();
Abstract Class
- An abstract class is a class that is incomplete & must be implemented in a derived class.
- A class can be marked as an abstract class by using the keyword “abstract” before the class definition.
- It can have methods with or without implementation.
- We cannot create an instance for an abstract class.
- A method inside an abstract class can be marked as abstract to force the derived classes to implement them.
- Eg:
public abstract class Hobby
{
private string name;
pubic string GetHobbyName()
{
return name;
}
public abstract string GetTypeOfHobby();
}
public class Photography : Hobby
{
public override string GetTypeOfHobby()
{
//implementation goes here...
}
}
How to prevent Polymorphism ?
- We can prevent a class from being derived by marking them as “sealed“.
- A sealed class cannot be a Base class.
- We can prevent a method from being overridden further by marking it as “sealed“.
NOTE: Only the overridden methods can be sealed.
When is Polymorphism required ?
- It is required with sealed classes.
- Performance improvement.
- Protecting the security measures.
NOTE: It is highly recommended to Seal a class or a method only if it is really needed, otherwise don’t.
Conclusion
Hope this article has helped you learn about Object Oriented Programming in a detailed and useful way.
We would love to receive your feedback and comments about our published articles. It motivates us to work towards making us more better and help you learn things in more details.
Do follow us at HDiWs.
Happy Learning.
Thank you.
Wow amazing blog layout How long have you been blogging for you made blogging look easy The overall look of your web site is magnificent as well as the content